Sharon
Liu
Habitat's Airlock Linkage System
Descending Light: Python Game
Fundamentals of Programming, Spring 2019
The objective of this term project was to combine various principles of programming: object-oriented programming, python packages and modules, functions, and pathfinding in order to create an interactive game. The project was heavily inspired by classic pixel horror games, particularly navigating mazes with zombies, and the style is reminiscent of that of Hotline Miami. The game contains 3 levels, which get progressively harder due to more enemies and more obstacles. The final level contains a final boss, which uses A-star pathfinding to find the closest route to the player. The main modules used in the python library are: pygame, pytmx, and pytweening. The files can be downloaded using this link.
Level 1 Gameplay
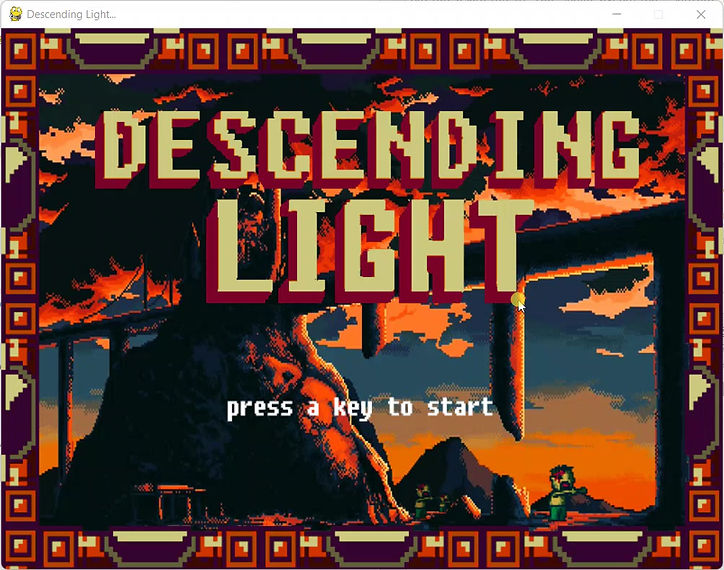
Level 1 contains 2 enemy types (normal and blocking zombies), and 1 weapon upgrade. Cockroaches and pizza are to restore health while coffee is to restore energy. After energy depletes to red, the player is largely slowed. The normal zombies use a direct radial path to the player and are relatively slow. The blocking zombies have greater health, but they traverse back and forth in one direction. Level 1 is the easiest map to navigate.
Level 2 Gameplay
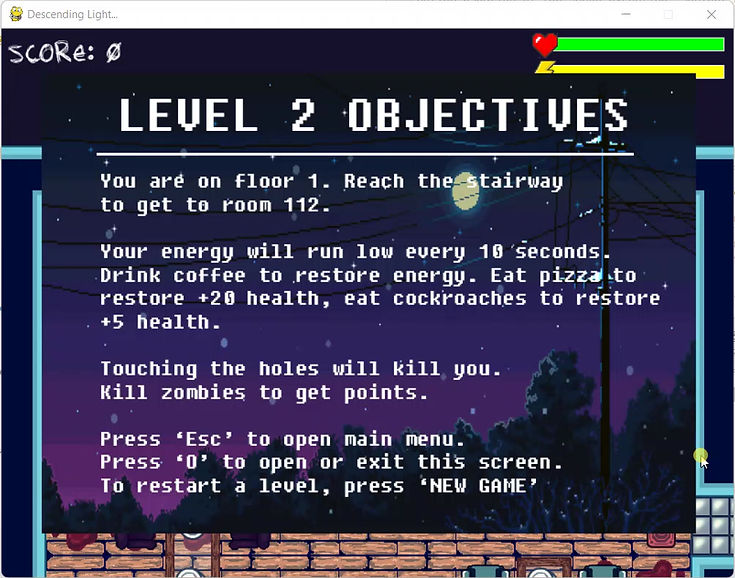
Level 2 contains 3 enemy types (normal, strong, and blocking zombies), along with holes that when the player touches will kill them. There is 1 weapon upgrade. Cockroaches and pizza are to restore health while coffee is to restore energy. After energy depletes to red, the player is largely slowed. The strong zombies are similar to normal zombies, but with greater speed and health. Level 2 is harder to navigate.
Final Level Gameplay

The final level has 1 enemy type (zombified monster). Though the map is smaller, the final boss is the hardest to beat. The sprite uses A-star path finding with weighted heuristics in order to find the closest route to the player at all times. If the player is trapped in a corner, it will be impossible to get out.
Storyboard and Concept
The list below highlights the timeline of progress throughout the duration of the project. Over the course of 3 weeks while meeting up with the term project mentor, I finished the check-ins and got approval for the project.
Timeline:
TP1 (Week 1): Implement player and enemy actions
-
Collision detection
-
Have 1 type of zombie appear; have it react to weapon collision and implement health bar
-
Have a makeshift weapon on the player (which will be a circle and rectangle until sprite sheets are created)
-
Include side-scrolling with a background (which will be updated)
TP2 (Week 2): Improve the UI and displays
-
Power-ups randomly dropped on floor
-
Scoreboard (potentially, or might remove)
-
Zombie randomly generates
-
When player health low, the screen flashes red (player/zombie collision)
-
Path finding algorithm
-
Sprite sheets (left/right, walking motion)
-
Map of Floor 2/ Floor 1 and rooms (also detects player location)
TP3 (Week 3): Polish graphics and audio
-
Playable character with correct movements
-
Graphics features: introduction story
-
Background, opening, closing, credits
-
Audio and music files
-
Include more item varieties
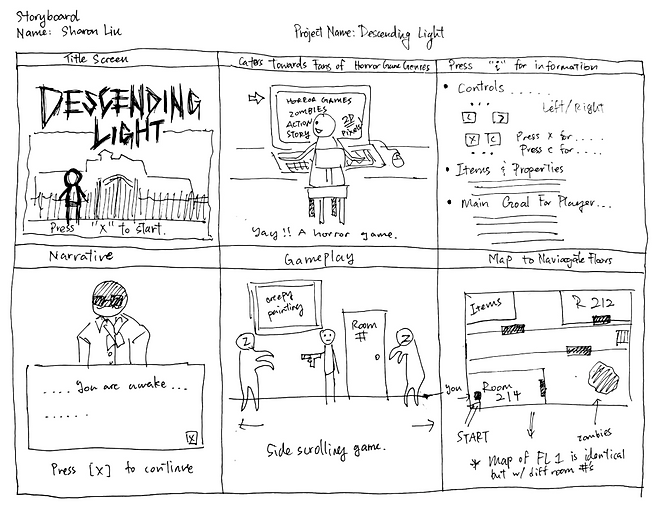
Initial storyboarding stage: incorporation of screens, side-scrolling, and basic UI and level designs
A* Pathfinding
Since the challenge of the project was to include a higher level concept of programming that was not taught in class, I had to research pathfinding. The boss in the game used A-star pathfinding, in which the boss sprite will always try to find the shortest route to the player along the map while generally avoiding the obstacles (chairs) in the room. The center of the sprite's hitbox is the "start" of the path, while the player is the "goal." The algorithm uses costs and estimates to determine the quickest and most efficient path. That being, f=g+h, where f is the total cost of the node in the tile map, g is the distance between the current and start node, and h is the heuristic or estimated distance from the current node to the goal. Since the sprite needs to move, it updates by following the position of appended list of the node path from the current node to the player.
General files and directory
Project Name: Descending Light
Run game using Game.py
Game Folder contains:
• Assets: music, sounds, score files
• Folders in same directory: images, maps
• 4 .py files:
- Game.py
- Constants.py
- TileMap.py
- AllSprites.py
Installation of following Python libraries:
• Python Library files (included folders):
- pygame
- pytmx
- pytweening
Controls:
• Main keys:
- Esc : main menu
- W/A/S/D : move char
- Q/E : rotate char ccw/cw
- Enter/Return : shoot
- M : toggle minimap
- O : Objectives screen
- P : Pause game